Search SWOT
This notebook explains how to search half orbits intersecting a geographical area.
To run this notebook you will need to download the swath geometries geojson files : Science phase geometries and Cal/Val phase geometries
Tutorial Objectives
Get the numbers of the half orbits intersecting with a geographical area.
Import + code
[1]:
import warnings
warnings.filterwarnings('ignore')
[2]:
import geopandas as gpd
from shapely import geometry
[3]:
import cartopy.crs as ccrs
import matplotlib.pyplot as plt
import matplotlib.patches as patches
[4]:
def get_half_orbits_intersect(bbox):
"""Get half orbits that intersect a bounding box.
Parameters
----------
bbox:
the bounding box
Returns
-------
gpd.GeoDataFrame:
A Geopandas dataframe containing intersecting half orbits numbers and geometries
"""
swath_geometries = gpd.read_file(GEOMETRIES_FILE)
bbox_polygon = geometry.box(*bbox)
def _filter_intersect(row, polygon):
half_orbit_polygon = row.geometry
return polygon.intersects(half_orbit_polygon)
select = swath_geometries.apply(_filter_intersect, polygon=bbox_polygon, axis=1)
return swath_geometries[select]
[5]:
def plot_geometries(geometries, lon_range, lat_range, title, plot_extent=None):
fig, ax = plt.subplots(ncols=1, figsize=(12, 8), subplot_kw={'projection': ccrs.PlateCarree()})
gpd.GeoSeries(geometries.geometry).plot(ax=ax,transform=ccrs.PlateCarree(),alpha=1)
square = patches.Rectangle((lon_range[0], lat_range[0]), lon_range[1]-lon_range[0], lat_range[1]-lat_range[0], edgecolor='orange', facecolor='none', transform=ccrs.PlateCarree())
ax.add_patch(square)
ax.set_title(title)
ax.coastlines()
if plot_extent:
ax.set_extent(plot_extent, crs=ccrs.PlateCarree())
Parameters
Define a geographical area
[6]:
# Mediterranean sea
lon_range = 6, 11
lat_range = 38, 43
[7]:
bbox = [lon_range[0], lat_range[0], lon_range[1], lat_range[1]]
plot_extent = [lon_range[0], lon_range[1], lat_range[0], lat_range[1]]
Define the phase
[8]:
# science, calval
phase = 'science'
Define the geometries file’s path to use
[9]:
GEOMETRIES_FILE = f'KaRIn_2kms_{phase}_geometries.geojson'
Search for matching half orbits
[10]:
swath_geoms = get_half_orbits_intersect(bbox)
[11]:
swath_geoms
[11]:
pass_number | geometry | |
---|---|---|
0 | 1 | POLYGON ((-83.37130 -77.05377, -82.41365 -77.0... |
28 | 29 | POLYGON ((-85.83706 -77.05377, -84.87940 -77.0... |
235 | 236 | POLYGON ((-65.48095 78.27207, -64.06949 78.268... |
250 | 251 | POLYGON ((-79.67267 -77.05377, -78.71502 -77.0... |
263 | 264 | POLYGON ((-67.94671 78.27207, -67.15265 78.270... |
278 | 279 | POLYGON ((-82.13843 -77.05377, -81.18077 -77.0... |
291 | 292 | POLYGON ((-70.41246 78.27207, -69.00100 78.268... |
306 | 307 | POLYGON ((-84.60418 -77.05377, -83.64652 -77.0... |
513 | 514 | POLYGON ((-64.24808 78.27207, -63.45402 78.270... |
541 | 542 | POLYGON ((-66.71383 78.27207, -65.30237 78.268... |
556 | 557 | POLYGON ((-80.90555 -77.05377, -79.94789 -77.0... |
569 | 570 | POLYGON ((-69.17958 78.27207, -67.76812 78.268... |
[12]:
half_orbits = list(swath_geoms['pass_number'])
half_orbits
[12]:
[1, 29, 236, 251, 264, 279, 292, 307, 514, 542, 557, 570]
Plot geometries
[13]:
plot_geometries(swath_geoms, lon_range, lat_range, f"Swot {phase} Geometries")
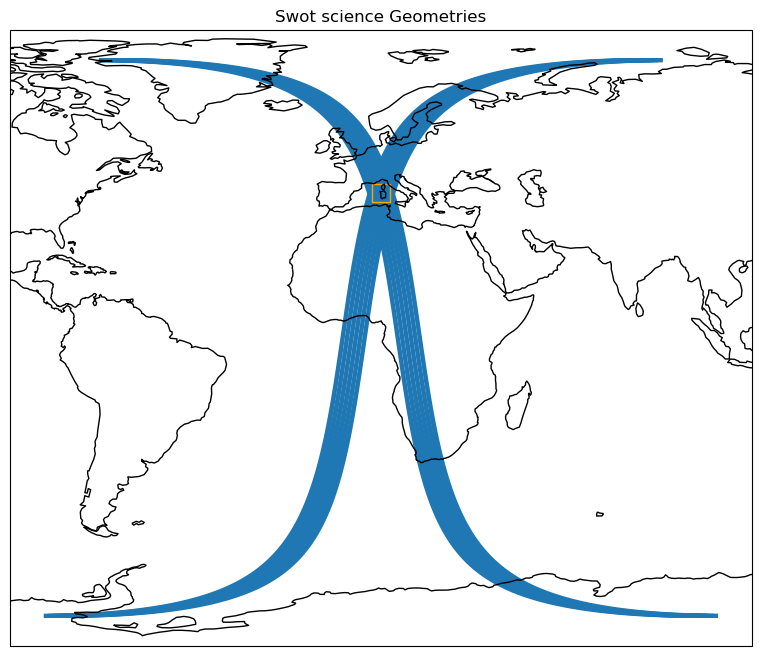
[14]:
half_orbit_num = 264
plot_geometries(swath_geoms[swath_geoms['pass_number']==half_orbit_num], lon_range, lat_range, f"Swot {phase} Geometry, half orbit={half_orbit_num}", plot_extent)
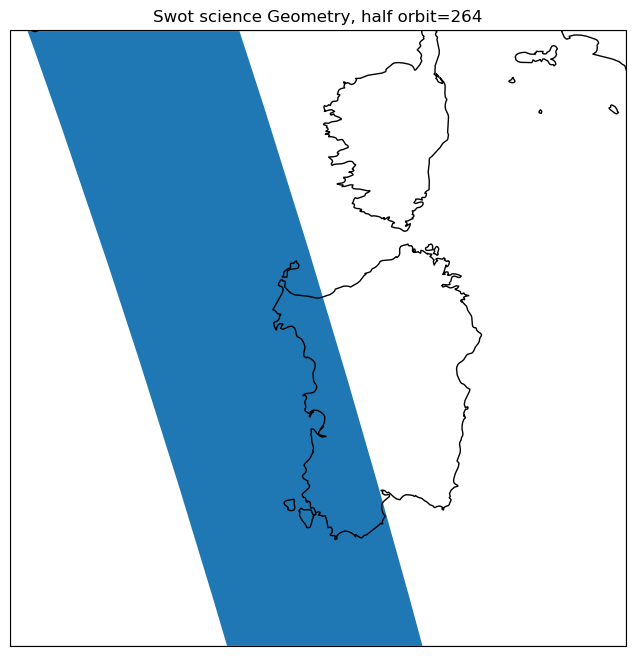